Description
Reads various data relating servo axis/spindle axis that is got by cnc_rdposition, cnc_rdspeed, cnc_rdsvmeter, cnc_rdspmeter, cnc_rdhndintrpt, with supporting "extended axis name".
You have to specify the argument "cls" with the data class to be read, array of short, "type[]" with the kind of data to be read. The number of the array must be specified by the argument "num". The data kind that can be read once is up to 4. If "num" exceeds 4, this function will return the error, EW_ATTRIB.
The argument "(*len)" should be specified with the number of axes to be read. According to "cls", "(*len)" should be specified as follows.
1. In case that "cls" is specified with Position(=1), Servo(=2), Spindle(=3):
2. In case that "cls" is specified with Selected spindle(=4), Speed(=5):
The read data will be stored on the array of ODBAXDATA structure specified by the argument "axdata[]". The number of members of this array must be "num" x "(*len)". The read data will be stored on the array, by the number of specified "(*len)" and order of specified "type[]" like as follows:
Data of type[0] | : | axdata[ | 0] | ,..,axdata[ | (*len)-1] |
Data of type[1] | : | axdata[ | (*len)] | ,..,axdata[ | 2 x (*len)-1] |
Data of type[2] | : | axdata[ | 2 x (*len)] | ,..,axdata[ | 3 x (*len)-1] |
Data of type[3] | : | axdata[ | 3 x (*len)] | ,..,axdata[ | 4 x (*len)-1] |
Even in case that specified "(*len)" is smaller than the actual number of axes, the data will be stored on the array according to the "(*len)" that was specified at calling of this function.
Example of arguments)
In case of reading Absolute position, Machine position, Relative position:
short types[3] = {0, 1, 2}; /* Absolute, Machine, Relative */
len = 3; /* Specify 3 axes */
ODBAXDT axdata[3*3];
cnc_rdaxisdata(h, 1, types, 3, &len, axdata);
The contents of the array "axdata" will be as follows.
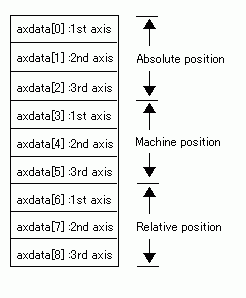
If the current effective number of axes is 2, (*len) will be returned 2 and array for 3rd axis(axdata[2], [5], [8]) will have no data after the execution.
MTConnect Fanuc AdapterUniversal Fanuc Driver
Fanuc Focas Library CD
Declaration
FWLIBAPI short WINAPI cnc_rdaxisdata(unsigned short FlibHndl, short cls, short* type, short num, short* len, ODBAXDT* axdata);
Arguments
Specify the library handle. See "Library handle" for details.
Specify the class of data to be read.
1 | : | Position value |
2 | : | Servo |
3 | : | Spindle |
4 | : | Selected spindle |
5 | : | Speed |
Specify the pointer for the array that specifies the kind of data to be read. The following value can be specified according to "cls".
- cls = 1 (Position value)
0 | : | Absolute position |
1 | : | Machine position |
2 | : | Relative position |
3 | : | Distance to go |
4 | : | Handle interruption(Input unit) |
5 | : | Handle interruption(Output unit) |
6 | : | Start point of program restart |
7 | : | Distance to go of program restart |
8 | : | Start point of block restart |
9 | : | Distance to go of block restart |
- cls = 2 (Servo)
0 | : | Servo load meter |
1 | : | Load current (% unit) |
2 | : | Load current (Ampere unit) |
- cls = 3 (Spindle) and cls = 4 (Selected spindle)
0 | : | Spindle load meter |
1 | : | Spindle motor speed |
2 | : | Spindle speed (according to parameter 3799#2) |
3 | : | Spindle speed (got from Spindle motor speed) |
4 | : | Spindle load meter (average of each 250ms) |
5 | : | Spindle load meter(maximum value) |
6 | : | Spindle load meter(maximum value)(average of each 250ms) |
7 | : | Time that spindle can continue processing |
? | Data by 5, 6, 7 will be influenced by parameter No.4542#7. So, the value of road meter (type = 0) might exceed maximum value (type = 5,6) depending on timing in which data is acquired. |
- cls = 5 (Speed)
0 | : | Feed rate(F)(Feed per minute) |
1 | : | Spindle speed(S) |
2 | : | Jog speed/Dry run speed |
3 | : | Tool tip speed |
4 | : | Rotation speed of servo motor |
5 | : | Feed rate(F/S) Note |
Note) | The unit follow the value of parameter No.3107#3 and No.3191#5. Judge the unit by "unit". |
Specify the number of array "type".
Specify the pointer that stores the number of axes to be read.
This function returns the actual read number of axes after the execution.
Specify the pointer of the array of ODBAXDT structure. Number of "num" x "(*len)" must be required. The ODBAXDT structure is as follows.
typedef struct odbaxdt {
char name[4]; /* axis name */
long data; /* data */
short dec; /* decimal point */
short unit; /* unit of data */
short flag; /* flags */
short reserve; /* reserved */
} ODBAXDT ;
- name
- Axis name will be stored in ASCII code.
The string is NULL terminated.
- data
- Data to be read
- dec
- Decimal point of data
In case that following "unit" is "rpm" or "%", this data is always 0.
- unit
- Unit of data
0 : mm (Position value) 1 : inch (Position value) 2 : degree (Position value) 3 : mm/minute (Feed rate(Feed per minute), jog/dry run speed, Tool tip speed) 4 : inch/minute (Feed rate(Feed per minute), jog/dry run speed, Tool tip speed)) 5 : rpm (Spindle speed, spindle motor speed) 6 : mm/round (Feed rate(Feed per revolution), jog/dry run speed, Tool tip speed)) 7 : inch/round (Feed rate(Feed per revolution), jog/dry run speed, Tool tip speed)) 8 : % (Load meter, load current) 9 : Ampere (Load current) 10 : Second (Time)
- flag
- Flags
According to "cls", following value will be set.
- cls = 1 (Position value)
bit 0 : Display state 1 = Displayed on CNC screen : 0 = Not displayed on CNC screen bit 1 : Axis detaching state 1 = enabled : 0 = disabled bit 2 : Interlock state 1 = enabled : 0 = disabled bit 3 : Machine lock state 1 = enabled : 0 = disabled bit 4 : Servo off state 1 = enabled : 0 = disabled bit 5 : In-position check 1 = Not in-position : 0 = In-position bit 6 : Mirror image state 1 = enabled : 0 = disabled bit 7 : Diameter and radius setting switching function 1 = switching : 0 = not switching bit 8 : High speed program check mode(only machine coordinate) 1 = enabled : 0 = disabled bit 9 : Optional one axis approach by program restart 1 = executing : 0 = not executing bit 10 : Restart coordinates display on the program restart screen(except machine coordinate) 1 = possible : 0 = impossible (display "***********") bit 11 : Release state of axis(Flexible path axis assignment function) 1 = enabled : 0 = disabled bit 12,..,15 : not used
- cls = 3, 4(Spindle, Selected spindle)
- ?type = 5,6,7(Spindle load meter(maximum value), Spindle load meter(maximum value)(average of each 250ms), Time that spindle can continue processing)
bit 0 : Data judgment result 1 = It has judged. : 0 = Unjudgment bit 1 : Presence of data 1 = Exist : 0 = None bit 12,..,15 : not used
- cls = 5 (Speed)
bit 0 : Spindle speed 1 = spindle exists : 0 = spindle not exist bit 1 : Jog speed/Dry run speed 1 = Dry run speed : 0 = Jog speed bit 2,..,15 : not used
- Others
not used
Return
EW_OK is returned on successful completion, otherwise any value except EW_OK is returned.
The major error codes are as follows.
Return code | Meaning/Error handling |
---|---|
EW_LENGTH (2) |
Data block error Number of axis(*len) is less or equal 0. |
EW_NUMBER (3) |
Data number error Data class(cls) is wrong. |
EW_ATTRIB (4) |
Data attribute error Kind of data(type) is wrong, or The number of kind(num) exceeds 4. |
EW_NOOPT (6) |
No option Required option to read data is not specified. |
As for the other return codes or the details, see "Return status of Data window function"
CNC option
And this function is related to the following CNC option.
The option that corresponds to the data to be read is required.
For HSSB connection,
The extended driver/library function is necessary.
For Ethernet connection,
The Ethernet function and the extended driver/library function are necessary.
However, in case of Series 0i-D/F, Series 30i and PMi-A, the required CNC option is as follows.
When Embedded Ethernet is used,
- above two optional functions are not required.
When Ethernet board is used,
- only Ethernet function is required.
CNC parameter
This function is related to the following CNC parameter.
See the manual of CNC parameter for details.
1020,1025,1026 | (influenced by setting) |
3131,3132,3133 | (influenced by setting) |
3104#0,#4,#5,#6,#7 | (influenced by setting) |
3115#0,#1,#2 | (influenced by setting) |
3799#2 | (influenced by setting) |
8163#0 | (influenced by setting) |
4542#7 | (influenced by setting) |
CNC mode
This function can be used in any CNC mode.
Available CNC
0i-A | 0i-B/C(Note) | 0i-D | 0i-F | 15 | 15i | 16 | 18 | 21 | 16i-A | 18i-A | 21i-A | 16i-B | 18i-B | 21i-B | 30i-A | 30i-B | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
M (Machining) | X | X | O | O | X | X | X | X | X | X | X | X | X | X | X | O | O |
T (Turning) | X | X | O | O | X | - | X | X | X | X | X | X | X | X | X | O | O |
LC (Loader) | - | - | - | - | - | - | X | X | X | X | X | X | X | X | X | - | - |
0i-D | 0i-F | 16i | 18i | 30i-A | 30i-B | |
---|---|---|---|---|---|---|
P (Punch press) | O | O | X | X | - | O |
L (Laser) | - | - | X | - | - | O |
W (Wire) | - | - | X | X | O | O |
Power Mate i-D | X |
Power Mate i-H | X |
Power Motion i-A | O |
"O" | : | Both Ethernet and HSSB | |
"E" | : | Ethernet | |
"H" | : | HSSB | |
"X" | : | Cannot be used | |
"-" | : | None |
Note) 0i-C does not support the HSSB function.
Example(C Language)
Absolute position, machine position, relative position, distance to go,
of all axes are got and displayed.
#include "fwlib32.h"
void example( void )
{
ODBAXDT pos[4*MAX_AXIS];
short types[4] = {0, 1, 2, 3};
short num = sizeof(types) / sizeof(types[0]);
short len = MAX_AXIS;
short ret = cnc_rdaxisdata(h, 1, types, 4, &len, pos);
if(!ret) {
int i;
printf("ABSOLUTE:\n");
for(i = 0 * MAX_AXIS ; i < 0 * MAX_AXIS + len ; i++) {
printf("%s = %d\n", pos[i].name, pos[i].data);
}
printf("MACHINE:\n");
for(i = 1 * MAX_AXIS ; i < 1 * MAX_AXIS + len ; i++) {
printf("%s = %d\n", pos[i].name, pos[i].data);
}
printf("RELATIVE:\n");
for(i = 2 * MAX_AXIS ; i < 2 * MAX_AXIS + len ; i++) {
printf("%s = %d\n", pos[i].name, pos[i].data);
}
printf("DISTANCE TO GO:\n");
for(i = 3 * MAX_AXIS ; i < 3 * MAX_AXIS + len ; i++) {
printf("%s = %d\n", pos[i].name, pos[i].data);
}
}
}
Example(C#)
Absolute position, machine position, relative position, distance to go,
of all axes are got and displayed.
class example
{
public void sample()
{
Focas1.ODBAXDT_data pos = new Focas1.ODBAXDT_data();
short[] types = { 0, 1, 2, 3 };
short len = Focas1.MAX_AXIS;
byte[] bytes = new byte[Marshal.SizeOf(pos) * 4 * Focas1.MAX_AXIS];
IntPtr ptrWork = Marshal.AllocCoTaskMem(Marshal.SizeOf(pos));
short ret = Focas1.cnc_rdaxisdata(h, 1, types, 4, ref len, bytes);
if (ret == Focas1.EW_OK)
{
int i;
Console.WriteLine("ABSOLUTE:");
for (i = 0 * Focas1.MAX_AXIS; i < 0 * Focas1.MAX_AXIS + len; i++)
{
Marshal.Copy(bytes, i * Marshal.SizeOf(pos), ptrWork, Marshal.SizeOf(pos));
Marshal.PtrToStructure(ptrWork, pos);
Console.WriteLine("{0} = {1}", pos.name, pos.data);
}
Console.WriteLine("MACHINE:");
for (i = 1 * Focas1.MAX_AXIS; i < 1 * Focas1.MAX_AXIS + len; i++)
{
Marshal.Copy(bytes, i * Marshal.SizeOf(pos), ptrWork, Marshal.SizeOf(pos));
Marshal.PtrToStructure(ptrWork, pos);
Console.WriteLine("{0} = {1}", pos.name, pos.data);
}
Console.WriteLine("RELATIVE:");
for (i = 2 * Focas1.MAX_AXIS; i < 2 * Focas1.MAX_AXIS + len; i++)
{
Marshal.Copy(bytes, i * Marshal.SizeOf(pos), ptrWork, Marshal.SizeOf(pos));
Marshal.PtrToStructure(ptrWork, pos);
Console.WriteLine("{0} = {1}", pos.name, pos.data);
}
Console.WriteLine("DISTANCE TO GO:");
for (i = 3 * Focas1.MAX_AXIS; i < 3 * Focas1.MAX_AXIS + len; i++)
{
Marshal.Copy(bytes, i * Marshal.SizeOf(pos), ptrWork, Marshal.SizeOf(pos));
Marshal.PtrToStructure(ptrWork, pos);
Console.WriteLine("{0} = {1}", pos.name, pos.data);
}
}
Marshal.FreeCoTaskMem(ptrWork);
}
}